CS120 Labs
Labs - All work is to be completed independently!
LAB1 - Command Line Compilation, the XCode IDE and XCode Debugging
Purpose: This lab will familiarize you with
compiling C++ programs from the command line, using the C++
XCode IDE environment, and using the XCode debugger tool. Please
note, this is the only lab that requires you to use XCode and
the T200 or Mac machines. For all other labs you may use either
the Xcode or Clion IDEs.
To login to the T200 machines the username is labuser and the
password is empty - this grants you permission to use XCode. Do
not login with your Wooster credentials.
PART1 : Create and compile your first
C++ program using the command line compiler.
- In order to run the following program, follow the instructions for command line compilation which can be found at this link. Copy and paste the following program into your Lab1.cpp file.
}/* PROJECT: Lab1 assignment on command line compilation FILE: main.cpp PURPOSE: To illustrate the use of command line compilation PROGRAMMER: Your Name
DATE:
REMARKS: */ #include <iostream> //include the input/output classes using namespace std; //use the standard C++ namespace so names do not have to be fully qualified int main() { cout << "Hello World!\n"; //print Hello World! to the terminal followed by a return return 0; //successful termination of main
Ask questions if you run into problems.
- Make the following changes to your first program, compiling
and executing after each modification.
- Comment out the "Hello World! output statement.
- Declare three integer variables with the initial value of 0.
- Read three integer values from the keyboard and store in
the integer variables declared in 2). Try to use cin instead of scanf - refer to the many online references (https://en.cppreference.com/w/).
- Print the three integer variable values of part 3) to the terminal with an appropriate string message. Try to use cout instead of printf - refer to the many online references.
- Compute and print the sum of the three integer variable
values of part 3) to the terminal with an appropriate string
message.
- Compute and print the average of the three integer variable values of part 3) to the terminal with an appropriate string message.
- Find and print the largest of the three integer variable values of part 3) to the terminal with an appropriate string message.
- Find and print the smallest of the three integer variable values of part 3) to the terminal with an appropriate string message.
Ask questions if you run into problems.
PART2: Using the XCode IDE
- Open the XCode IDE which can be found in the Doc - a hammer on
a blue background. Follow the directions for creating a simple
XCode C++ project found at this link and
- Name your project as PascalsTriangle.
- Name your source file as main.cpp. Use the following program for this part of the lab (note that this program will also be used in PARTs 3 and 4 of the lab). You can simply paste the program code into your main.cpp file when you reach that step in the tutorial. Read the code carefully and try to determine the purpose of each function and how it is working.
/* PROJECT: Lab assignment on debugging FILE: main.cpp PURPOSE: To compute Pascal's triangle and illustrate the use of the debugger. PROGRAMMER: Denise Byrnes REMARKS: The code below is chosen to illustrate the debugger. It is neither the most efficient nor the most reliable way to solve this problem. */ #include <iostream> #include <iomanip> using namespace std; /*------------------------------------------------------------------*/ void skip(int n) /* PURPOSE: To skip n spaces on a line RECEIVES: n - the number of spaces to skip REMARKS: n should be non-negative */ { int i; /* a counter */ for (i = 0; i <= n; i++ ) cout << " "; } /*------------------------------------------------------------------*/ int factorial(int n) /* PURPOSE: To calculate n factorial RECEIVES: n - calculate the factorial of n RETURNS: n factorial REMARKS: n must be >= 0. Also if n is too large overflow may result */ { int product; /* accumulator for the running product */ int i; /* a counter */ product = 1; for (i = 1; i <= n; i++) { product = product + i ; } return(product); } /*------------------------------------------------------------------*/ int combination(int n, int k) /* PURPOSE: to calculate the number of combinations of n things taken k at a time (n choose k) RECEIVES: n - the number of items to choose from k - the number of items choosen RETURNS: n choose k REMARKS: n and k must be non-negative and k <= n. This program uses the formula (n choose k) = n! / ( k! * (n-k)! ). */ { int comb = factorial(n) / factorial(k) * factorial(n-k); return comb; } /*------------------------------------------------------------------*/ int main() { int nrows; /* the number of rows to print */ int n; /* a counter for the current row */ int k; /* a counter for the current column */ int comb; /* the number of combinations */ int spaces_to_skip; /* spaces to skip */ cout << "Enter the number of rows (<=13) in Pascal's triangle: "; cin >> nrows; cout << "\n\n\n"; /* print the title * / skip(16); cout << "TABLE 1: THE FIRST " << nrows << " ROWS OF PASCAL'S TRIANGLE\n\n"; / * start a loop over the number of rows */ spaces_to_skip = 36; for (n = 0; n < nrows; n = n + 2) { skip(spaces_to_skip); /* space to make a triangle */ spaces_to_skip = spaces_to_skip - 2; for (k = 0; k <= n; k++) { comb = combination(n,k); cout << setw(4) << comb; } cout << "\n" ; } return 0; }
- Under the "Product" menu select "Run" to execute the program and enter the integer 5 at the console when prompted for the number of rows to display in the triangle. The console should appear in the lower middle-right window. If you can not see the console then go to the "View" menu and select "Debug Area" and "Activate Console". You might have to resize the console window to see the output in "Triangle" form. Ignore any logic errors you discover when running the program. These errors are addressed in PART4 of the lab.
Ask questions if you run into problems.
PART3: Using the XCode Debugger.
Download and print this answer sheet
for PART3 and PART4 of the lab
- Read this quick little tutor on the XCode debugger. Read just the short "Debugger" section.
- Add a breakpoint in PascalsTriangle.cpp at the cin statement in main by clicking on the line number of the statement.
- Run the program and you should see a window similar to the following,
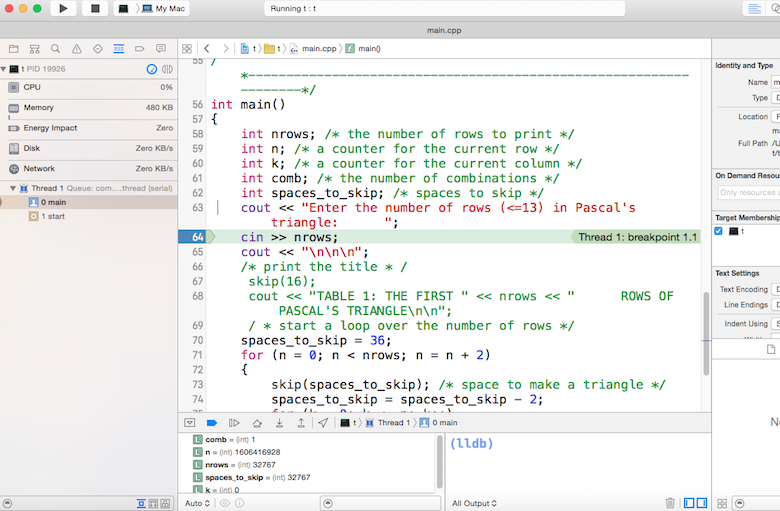
- The right pane shows the currently executing functions
(interrupted at this point because of the "Break point"). The
middle pane shows the statement that caused the interrupt -
marked in green, and the middle/bottom-left pane shows the
variables local to main and their default (garbage) values.
- Select the "Debug" menu and examine the
options you have while in debug mode - these are the top options above first
horizontal line. Try each of these options and determine what
each does. You may have to stop and restart your program -
stop a running program using the "Stop" option under the
"Product" menu.
- Select "Step Over" in the debugger to see the prompt "Enter the number of rows (< 13) in Pascal's triangle: " in the console window. Type in the integer 5 in the console window. Now check the value of nrows in the variables window of the debugger - it should equal 5.
- Select "Continue" in the debugger
and you should see the same output in the console as was
produced during PART2 of the lab.
Ask questions if you run into problems.
PART4: Debugging logic errors in the Pascal's triangle program
Print and answer all questions on the Debugger.doc handout and turn in before leaving the lab.
The program's purpose is to display Pascal's Triangle, a sequence of integers that arises in numerous areas of math and computer science, especially combinatorics and probability. When completed, the program's output should be:
Enter the number of rows (<= 13) in Pascal's Triangle. THE FIRST 5 ROWS OF PASCAL's TRIANGLE: 1 1 1 1 2 1 1 3 3 1 1 4 6 4 1
Entries in Pascal's triangle are indexed by integers. n is the number of the row and k is the position from the leftmost member of the row. The indices in both directions start at zero (0), so in the fifth row listed above, C(4,0) = 1, C(4,1) = 4, and so on.
The values themselves are computed by the formula C(n, k) = n! / ( k! (n-k)!), which is called a combination. n! denotes a factorial, that is, the product n(n-1)(n-2)...(2)(1). The combinations can be interpreted as the number of ways to choose k elements from a collection containing n elements. When described, it is customary to say "n choose k", for instance '4 choose 3 is 4' ".
Answer question 1 on Debugger.doc handout.
As you will discover with the help of your debugger, the program to compute Pascal's triangle has some mistakes. Each provides an opportunity to learn a debugging concept in context of it's use.
Answer question 2 on Debugger.doc handout.
Step over / inspect
- Use the Stop command to reset your debugger.
- Remove the breakpoints used in PART3 of the lab.
- Add a breakpoint at the first cout statement in main.cpp.
- Use the Run command to restart the program from the very beginning.
- Now use the Step Over command, until the active line
is
cin >> nrows;
- Select Step Over until the program is now expecting keyboard input because the cin >> nrows line is being executed.
- Type 5 (followed by the Enter key) for the input.
Answer question 3 on Debugger.doc handout.
- Inspect the variables window.
- Inspect the value of nrows.
Answer question 4 on Debugger.doc handout.
More stepping
- Select Continue once
- Look at the output for Pascal's triangle.
Answer question 5 on Debugger.doc handout.
That is strange. The program is supposed to print three newlines, then the title. Let's find out why the title doesn't appear.
- Select Stop to start the debugger once again.
- elect Step Over until you reach the line prompting for input.
- Enter 5 for nrows
- Select Step Over one more time.
Answer question 6 on Debugger.doc handout.
- Notice that you skipped over several lines, including the line
cout << "TABLE 1: THE FIRST " << nrows << " ROWS OF PASCAL'S TRIANGLE\n\n");
The reason is that two delimiters for comments had extra spaces in them. - Select Stop to start the debugger once again.
- Change the line
/* print the title * / into
/* print the title */
and the line
/ * start a loop over the number of rows */ into
/* start a loop over the number of rows */ - Save your changes.
Answer question 7 on Debugger.doc handout.
Variables Window
- Select Run to run the program. Use Continue in the debugger to see output for questions 8 and 9.
- Enter 5 for the number of rows. (Use 5 for input for all the runs until part 11, below).
Answer question 8 on Debugger.doc handout.
Answer question 9 on Debugger.doc handout.
- To find out why there are not five rows, return to the debugger and select the Run command.
- Select Step Over until you reach the line for (k = 0; k <= n; k++) - before first iteration of inner loop.
Answer question 10 on Debugger.doc handout.
- Keep selecting Step Over until you at the line cout << "\n" ; - after completing one iteration of the outer loop.
Answer question 11 on Debugger.doc handout.
- Keep selecting Step Over until you exit the for loops and again arrive at the line cout << "\n";
Answer question 12 on Debugger.doc handout.
- View the program output. Notice that there are only three rows in the triangle. This is because the n values listed above went from 0 to 2 to 4 to 6. That is, the loop index i should have been increased by one each time, not two.
- Select Stop to end the debugger session.
- Change the line of code: for (n = 0; n < nrows; n = n + 2) to be for (n = 0; n < nrows; n++)
- Save your changes.
- Select Run and input 5 to the program where prompted. You should now have five rows in the triangle.
Step into
- The entries in the triangle are still incorrect. To see one
reason why, Debug and press Step Over quite a
few times, until the variable window first displays:
n: 2 k: 0
You should be at line comb = combination(n, k); - Press Step Into, to get to the line int combination = factorial(n) / factorial(k) * factorial(n-k); Notice that Step Into goes into each function as it is executed. That is why we've been using it to start the main() program from the very beginning. Using Step Over always steps over the next function to the next line of code in the current procedure.
- Press Step Into again. You should be at the line int product = 1; in the function factorial(n)
- Now press Step Over until you are at the line return product;
Answer question 13 on Debugger.doc handout. Note that k is undefined in the variables window since k is not active in the function factorial.
Answer question 14 on Debugger.doc handout.
- The reason that product in the inspect window is
incorrect is that the line
product = product + i;
should beproduct = product * i;
- Stop the debugger.
- Make the above change in your editor.
- Save your program.
- Run the program again with input of 5. Oh no! the triangle is still wrong!
Setting breakpoints
It is tedious to have to keep stepping to get inside a function. A more efficient way is to set a breakpoint at a line of interest. Then the program runs at full speed until a breakpoint is reached.
- Remove all breakpoints. Select the line comb = combination(n,k) ; in main and add a
breakpoint.
- Select Run - the program stops at the breakpoint. Don't forget to enter 5 in the terminal window for the number of rows
- Repeat selecting Continue until the variables window
displays
n: 3 k: 1
- Then Step Into once
- and then Step Over until you are at the line: return comb;
- Inspect the value of comb in the variables window
Answer question 15 on Debugger.doc handout.
- The true value for the combination of 3 things taken 1 at a time is 3! / 1!·2! = 6 / 2 = 3. So, the above value of comb is wrong.
- Something must be wrong with our combination calculation. In
fact, the programmer has ommitted parentheses in the denominator
for the line:
comb = factorial(n) / factorial(k) * factorial(n-k) ;
- Terminate the debugger.
- Change the line to be:
comb = factorial(n) / (factorial(k) * factorial(n-k)) ;
- Save your change and Run
Answer question 16 on Debugger.doc handout.
Finally
- Select the line comb = combination(n,k) ;
- Remove the Breakpoint.
- Select Run
Answer question 17 on Debugger.doc handout.
Submit a Folder: You should upload a "CS120Lab1YourName" zipped folder created for this lab to moodle. It must contain the Lab1.cpp program from Part1 and your corrected Pascal's Triangle program from Part4.
- Hand in, to the Instructor or TA, a hardcopy of the Debugger.doc question sheet with your answers before leaving the lab.
Graded on: Correct answers on Debugger.doc provided to TA, correct
Lab1.cpp program from PART1, correct Pascal's Triangle program
from PART4, and correct electronic drop. BE SURE TO ADD YOUR NAME TO ALL source files you create or modify!!!
LAB2 - Black Box and White Box program testing
Purpose: This lab will familiarize you with
basic debugging techniques that work in all development
environments and are independent of specific testing and debugging
tools. Make sure you have completed the reading for lab 2, http://softwaretestingfundamentals.com/white-box-testing/
PART1 : Create and compile the C++ program for this lab.
- Download and print pages 3-7 of the manual handout found at this link.
- Open your IDE, which can be found in the Docs menu.
- Create a C++ command line Project
- Save the project on the Desktop.
- Name your project CS120Lab2YourName
- Create the proper source file, named search.cpp.
- Use the following program for this lab. You can simply paste the program code into your search.cpp file, overwriting all existing text in the window, or download the file.
/*--- search.cpp -----------------------------------------------------
Program to read a 3 X 3 matrix of integers mat and an integer item,
and search mat to see if it contains item.
Add your name here and other info requested by your instructor.
--------------------------------------------------------------------*/ #include <iostream>
using namespace std;
const int SIZE = 3; // Set matrix size
typedef int Matrix[SIZE][SIZE]; // Define data type Matrix
bool matrixSearch(Matrix & mat, int n, int item);
/*---------------------------------------------------------------------
Search the n X n matrix mat in rowwise order for item.
Precondition: Matrix mat is an n X n matrix of integers with n > 0.
Postcondition: True is returned if item is found in mat, else false.
---------------------------------------------------------------------*/
int main()
{
// Enter the matrix
Matrix mat;
cout << "Enter the elements of the " << SIZE << " X " << SIZE
<< " matrix rowwise:\n";
for (int i = 0; i < SIZE; i++)
for (int j = 0; j < SIZE; j++)
cin >> mat[i][j];
// Search mat for various items
int itemToFind;
char response;
do
{
cout << "Enter integer to search for: ";
cin >> itemToFind;
if (matrixSearch(mat, SIZE, itemToFind))
cout << "item found\n";
else
cout << "item not found\n";
cout << "\nMore items to search for (Y or N)? ";
cin >> response;
}
while (response == 'Y' || response == 'y');
}
//-- (-- Incorrect --) Definition of matrixSearch()
bool matrixSearch(Matrix & mat, int n, int item)
/*--------------------------------------------------------------------
Search the n X n matrix mat in rowwise order for item
Precondition: Matrix mat is an n X n matrix of integers with n > 0.
Postcondition: True is returned if item is found in mat, else false.
NOTE: mat[row][col] denotes the entry of the matrix in the
(horizontal) row numbered row (counting from 0) and the
(vertical) column numbered col.
-------------------------------------------------------------------*/
{
bool found;
for (int row = 0; row < n; row++)
for (int col = 0; col < n; col++)
if (mat[row][col] == item)
found = true;
else
found = false;
return found;
}
- Compile and run the program using the "Run" option under the Product menu. Be sure to activate the console window.
- Enter the appropriate values at the console when prompted. You will probably find it most convenient to type three values, separated by space and ending with an Enter for each of the three rows in the matrix. Ignore any logic errors (like an incorrect search). These errors are addressed later in the lab. As you experiment with searching, try searching for the last value entered.
PART2 : Work with white-box and black-box testing
In the lab manual pages, complete the activities for Lab 1.1
- In the traces of items 6 and 7, think of each row of the trace as describing the status of the program before the corresponding line of code executes.
- In item 10, the author wants you to decide what code change is appropriate and make it in your actual code and then explain why it is more efficient in the sense that it has the potential to execute more quickly.
PART3 : Submit your completed lab
- Submit your zipped project folder via moodle and annotated with
CS120Lab2YourName.
- Hand in the lab manual pages for Lab1.1 to the TA.
Graded on : Correct answers in lab manual, correct search.cpp program file, and correct moodle submission.
Purpose: The purpose of this lab is to explore the implementation and applications of a Text ADT, a simplified version of the STL’s string class.
Please download the starting packet, Lab3.zip, for this lab.
This folder contains
- config.h - a header file used to turn certain debugging tests on and off
- LexicalAnalyzer.cpp - a stub program you must complete for the in-lab exercise
- progsamp.dat - the input file for LexicalAnalyzer.cpp
- subjects.txt - ignore
- test1.cpp - the test driver for your implementation of the Text ADT
- Text Worksheets.pdf - print this file and provide answers for the testing scenarios in the document. A hard copy of the worksheets must be turned into the TA by the end of the lab session.
- Text_ADT.doc - clarification for the Lab3 assignment, some notes and hints (code segments)
- Text.h - starting header file for the Text ADT. Completing the implementation of Text.cpp is the prelab exercise and you should start on this before the lab session
- textio.cpp - code stubs for certain methods used in the lab
- Text ADT Manual pages.pdf - the manual pages for the assignment
Refer to the lab manual pages provided as a handout and Text_ADT.doc for directions on completing the lab.
- Submit your source code and input files via moodle in a zipped folder
annotated with CS120Lab3YourName.
- Hand in your lab worksheets and grade sheet to the TA.
Graded on : Correct answers in lab worksheets provided to TA, grading sheet provided to TA, correct source files (all prelab and inlab files with input files provided) submitted to moodle.
Purpose: The purpose of this lab is to explore the implementation and applications of a BlogEntryADT, which is built on the Date class and the Text ADT class of Lab3.
Please download the starting packet, Lab4.zip, for this lab. This folder contains
- config.h - a header file used to turn certain debugging tests on and off
- test2.cpp - the test driver for your implementation of the BlogEntry ADT
- BlogEntry Worksheets.pdf - print this file and provide answers for the testing scenarios in the document. A hard copy of the worksheets must be turned into the TA by the end of the lab session.
- BlogEntry ADT.doc - clarification for the Lab4 assignment, some notes and hints (code segments)
- BlogEntry.h - starting header file for the BlogEntry ADT. Completing the implementation of BlogEntry.cpp is the prelab exercise and you should start on this before the lab session.
- Date.h - starting header file for the Date ADT. Completing the implementation of Date.cpp is the prelab exercise and you should start on this before the lab session.
- Text.h - header file for Text.h from lab3, use your own implementation of Text.cpp from lab3.
- show2.cpp - code stubs to display a Date object and a BlogEntry object
- Blog ADT Manual pages.pdf - the manual pages for the assignment
Refer to the lab manual pages provided as a handout and BlogEntry ADT.doc for directions on completing the lab.
- Submit your source code and input files via moodle in a zipped folder
annotated with CS120Lab4YourName.
- Hand in your lab worksheets and grade sheet to the TA.
Graded on : Correct answers in lab worksheets provided to TA, grading sheet provided to TA, correct source files (all prelab and inlab files with input files provided) submitted to moodle.
- config.h - a header file used to turn certain debugging tests on and off
- Array List ADT.doc - clarification for the assignment, some notes and hints (code segments)
- List Worksheets.pdf - print this file and provide answers for the testing scenarios in the document. A hard copy of the worksheets must be turned into the TA by the end of the lab session.
- ListArray.h - starting header file for the List ADT. Completing the implementation of ListArray.cpp is the prelab exercise and you should start on this before the lab session.
- show3.cpp - code to complete the implementation of the method to display the List Array
- sumintegers.cpp - a testing stub for one of the test cases discussed in the manual
- test3.cpp - the test driver for your implementation of the List ADT
- test3dna.cpp - a stub program you must complete for the in-lab exercise
- ArrayList ADT Manual pages.pdf - the manual pages for the assignment
Refer to the lab manual pages (Lab3) provided as a handout and Array List_ADT.doc for directions on completing the lab.
- Submit your source code and input files via moodle in a zipped folder
annotated with CS120Lab6YourName.
- Hand in your lab worksheets and grade sheet to the TA.
Graded on : Correct answers in lab worksheets provided to TA, grading sheet provided to TA, correct source files (ALL prelab and inlab files with input files provided) submitted to moodle.
Purpose: The purpose of this lab is to explore the implementation and applications of an ordered array-based List ADT, using C++'s inheritance and template mechanism.
Please download the starting packet, Lab6.zip, for this lab. This folder contains
- Ordered List ADT.doc - clarification for the assignment, some notes, hints (code segments), etc.
- Ordered List Manual Pages.pdf - the manual pages for the assignment
- Ordered List worksheets.pdf - print this file and provide answers for the testing scenarios in the document. A hard copy of the worksheets must be turned into the TA by the end of the lab session.
- config.h - a header file used to turn debugging tests on and off
- lab04-ex1.cpp - a sample test program from the lab manual
- ListArray.h - header file for the Array List ADT completed in lab 5. This file contains the implementation for the Array List of Lab4 - please use this file as it has compiler directives that handle printing packet contents.
- OrderedList.h - header file for the Ordered List ADT
- show4.cpp - code to complete the implementation of the method to display the Ordered List
- packet.cs - a stub program for the in lab exercise
- test4.cpp - the test driver for your implementation of the Ordered List ADT
- search.cpp - a stub program for searching an ordered list
- message.dat - an input file for the in lab exercise
Refer to the provided lab manual pages (Lab4) and Array List ADT.doc for directions on completing the lab.
- Submit your source code and input files via moodle in a zipped folder
annotated with CS120Lab6YourName.
- Hand in your lab worksheets and grade sheet to the TA.
Graded on : Correct answers in lab worksheets provided to TA, grading sheet provided to TA, correct source files (ALL prelab and inlab files with input files provided) submitted to moodle.
LAB7 - Linked List ADT
Purpose: The purpose of this lab is to explore the
implementation and applications of an linked one-way List ADT, using C++'s encapsulation and
template mechanism.
Please download the starting packet, Lab7.zip, for this lab. This folder contains
- Linked List Impl ADT.doc - clarification for the assignment, some notes, hints (code segments), etc.
- Lab7 Manual Pages.pdf - the manual pages for the assignment
- Lab7worksheets.pdf - print this file and provide answers for the testing scenarios in the document. A hard copy of the worksheets must be turned into the TA by the end of the lab session.
- config.h - a header file used to turn debugging tests on and off
- LinkedList.h
- header file for a linked List ADT.
- show5.cpp - code to complete the implementation of the method to display the Linked List
- slideshow.cpp - a stub program for the postlab exercise
- test5.cpp - the test driver for your implementation of the Linked List ADT
- slides.dat - an input file for the postlab exercise
Refer to the provided lab manual pages (Lab5) and Linked List Impl ADT.doc for directions on completing the lab.
- Submit your source code and input files via moodle in a zipped folder
annotated with CS120Lab7YourName.
- Hand in your lab worksheets and grade sheet to the TA.
Graded on : Correct answers in lab worksheets provided to TA, grading sheet provided to TA, correct source files (all prelab and inlab files with input files provided) submitted to moodle.
Purpose: The purpose of this lab is to explore the implementation and applications of a Stack ADT, using C++'s encapsulation and template mechanism. Two implementations, stack as array and stack as a list, are required.
Please download the starting packet, Lab8.zip, for this lab. This folder contains
- Stack ADT.doc - clarification for the assignment, some notes, hints (code segments), etc.
- Stack Manual Pages.pdf - the manual pages for the assignment
- Stack Worksheets.pdf - print this file and provide answers for the testing scenarios in the document. A hard copy of the worksheets must be turned into the TA by the end of the lab session.
- config.h - a header file used to turn debugging tests on and off
- Stack.h, StackArray.h, StackLinked.h
- header files for the Stack ADT.
- show6.cpp - code to complete the implementation of the method to display the Stack
- delimiters.cs - a stub program for the postlab exercise
- test6.cpp - the test driver for your implementation of the Stack ADT
Refer to the provided lab manual pages (Lab6) and Stack ADT.doc for directions on completing the lab.
- Submit your source code and input files via moodle in a zipped folder
annotated with CS120Lab8YourName.
- Hand in your lab worksheets and grade sheet to the TA.
Graded on : Correct answers in lab worksheets provided to TA, grading sheet provided to TA, correct source files (all prelab and inlab files with input files provided) submitted to moodle.
Purpose: The purpose of this lab is to perform a timing analysis of several provided algorithms using your implementation of a Timer ADT
Please download the starting packet, Lab9.zip, for this lab. This folder contains
- Lab 9 Performance Evaluation.doc- clarification for the assignment, some notes, hints (code segments), etc.
- Performance Evaluation Manual Pages.pdf - the manual pages for the assignment
- Performance Evaluation Worksheets.pdf - print this file and provide answers for the testing scenarios in the document. A hard copy of the worksheets must be turned into the TA by the end of the lab session.
- config.h - a header file used to turn tests on and off for the Timer ADT
- Timer.h - header file for the Timer ADT.
- search.cpp - code segment for evaluation of searching
- sort.cpp - code segment for evaluation of sorting
- test13.cpp - the test driver for your implementation of the Timer ADT
Refer to the provided lab manual pages (Lab13) and Lab 9 Performance Evaluation.doc for directions on completing the lab.
- Submit your source code and input files via moodle in a zipped folder
annotated with CS120Lab9YourName.
- Hand in your lab worksheets and grade sheet to the TA.
Graded on : Correct answers in lab worksheets provided to TA, grading sheet provided to TA, correct source files (all prelab and inlab files with input files provided) submitted to moodle.
Purpose: The purpose of this lab is to explore the implementation and applications of a Queue ADT, using C++'s encapsulation and template mechanism. Two implementations, queue as array and queue as a list, are required.
Please download the starting packet, Lab10.zip, for this lab. This folder contains
- 10-Queue ADT.doc - clarification for the assignment, some notes, hints (code segments), etc.
- Lab10 Manual Pages.pdf - the manual pages for the assignment
- Lab10 Worksheets.pdf - print this file and provide answers for the testing scenarios in the document. A hard copy of the worksheets must be turned into the TA by the end of the lab session.
- config.h - a header file used to turn debugging tests on and off
- Queue.h, QueueArray.h, QueueLinked.h
- header files for the QueueADT.
- show7.cpp - code to complete the implementation of the method to display the Queue
- storesim.cs - a stub program for the postlab exercise
- test7.cpp - the test driver for your implementation of the Queue ADT
Refer to the provided lab manual pages (Lab7) and 10-Queue ADT.doc for directions on completing the lab.
- Submit your source code and input files via moodle in a zipped folder
annotated with CS120Lab10YourName.
- Hand in your lab worksheets and grade sheet to the TA.
Graded on : Correct answers in lab worksheets provided to TA, grading sheet provided to TA, correct source files (all prelab and inlab files with input files provided) submitted to moodle.
LAB11 - Chained Hash Table with STL vector and list
Implementing a Hash Table class, pages 87-89 in the provided lab manual pages. Download the Lab11 input file here.
- You may use the STL vector class or a statically allocated array for your Hash Table and chained collisions must be implemented using the STL list class.
- For Step A4: Instead of adding a Display method to the HashTable class, overload the operator<<() free function as a friend of the class.
- You must write your own header and class implementation files complete with pre and post condition documentation. The files must be named HashTable.h and HashTable.cpp
- You must write a simple main test driver, named main.cpp. Once that is working you should process the provided input file using your Hash Table and producing the output shown below,
enter a file name : inputTest11.txt
Here is your hashtable :
Index 0 : SINCERELY THAT AZORES
Index 1 : BUT CAMELS
Index 2 : BIG ABOUT
Index 3 : AND AND AND AND AND JIM AND MARLIN
Index 4 : SMALL THE WERE THE AARDVARKS THE
Index 5 :
Index 6 : ANTS ANTS
Index 7 : BATS BATA PS TO SHIPPED DEAR
Index 8 : IN ARE CATS ARE ARE ANIMALS ARE CATS
Index 9 :
Index 10 : SORRY
Index 11 : BETWEEN BETWEEN
Index 12 : COWS COWS MISTAKENLY
- Submit your source code files via moodle in a zipped folder annotated with CS120Lab11YourName.
- Hand in the Project grade sheet to the TA.
- FOR BONUS POINTS; implement additional methods such as isKeyInTable(key), retrieveKey(key), removeKey(key), printCollisons(int i), which would print a list of items in the table at index i. Be sure to add tests for these to your main.cpp.
Graded on : Correct answers in lab worksheets provided to TA, grading sheet provided to TA, correct source files (inlab files with input files provided) submitted to moodle.