Final Project (100 pts)
Choose a problem to solve using Python programs.
You will work in teams of 3 students.
Your group will present the problem, your coded solution, and your results.
This project will be completed in stages.
-
Complete the survey on Moodle to be placed in a team. Note that you’ll work in your team for this final project as well as all in-class group activities.
- [10] Project proposal: Each team will submit to Moodle the following
- the title of your project,
- all team members, and
- one paragraph description of your project.
-
[20] Intermediate results: share with the class the current state of your project. It should run and produce some output, but it doesn’t need to be complete.
- [30] Source code: Submit your software in Moodle. Keep it well organized, use functions, and document your code using comments. Part of the code must be written by you - not just code from the textbook or from another source. If you use a resource you must cite it! Otherwise, it is plagiarism and will result in an F. Your source code should have:
- Comments with names of all team members at the top of the file
- One paragraph per team member describing their main contribution
- Comments at the top of each function
- Several functions (at least 3, but you will likely have more)
- Good use of variables and choice of variable names
- Conditional statements (if, for, while)
- [30] Presentation: four (4) powerpoint slides presented in class (~5 minutes per team) and submitted to Moodle. Your presentation should include:
- 1 slide: title, team names, class with semester/year
- 1 slide: present the problem
- 1 slide: present the solution (code, diagram, or a few bullets). Be clear what each team member’s main contribution is.
- 1 slide: results (pictures, graphs, numbers)
- A demo of your software
- [10] Peer grade: feedback from your peers on your project and how well you collaborated.
Grading
You will be graded on the above criteria as well as:
- Timely and correct submissions
- Working software - I should be able to run it successfully
- Originality - there should be some original code apart from possible start up code from the book/internet
- Grammar, content, formatting, correct citations, presentation, collaboration
Ideas
You are free to solve any problem, but here are some possible ideas with corresponding details on where you can look to get started.
- Turtle application (ch 1)
- Fractals (ch 9)
- Ex: Create a menu that asks you to draw sierpinsky triangle, rectangle, square, etc.
- Ex: Use recursion to create snowflakes, ferns-like shapes, or other interesting patterns
- Solar system (ch 10)
- Ex: Make it to be a real solar system
- Ex: Add a moon to the earth
- Image processing (ch 6)
- Ex: Create an image/landscape using cImage.py class
- Ex: Import an image of yourself and change its background
- Ex: Extend edge detection
- Ex: Flip the image
- Encryption/Decryption application (ch 3 and ch 8)
- Ex: Use regular expressions to crack a code or find genes in DNA string
- Ex: Implement other encryption/decryption algorithm that is not in the textbook
- Ex: Steganography (encode messages in a picture)
- Compute and plot some statistics for data downloaded from the web (ch 4)
- Data mining, clustring (ch 7)
- Predator-prey simulation (ch 11)
- Video game (ch 13)
- Ex: Rock, paper, scissors game (use TKinter to draw 3 corresponding buttons)
- Lottery ticket simulation
- Blackjack, poker, tic-tac-toe
- Hangman (use cImage to draw head/neck/etc)
Additional References
- The Python Standard Library, which includes many other packages that can be imported and used. No installation is required. For example,
tkinter
provides a graphical user interface,
- Turtle
Projects from previous years
Here is a sample of projects from 2020.
Previous years also included:
Games - battleship, blockout, hangman, space invaders
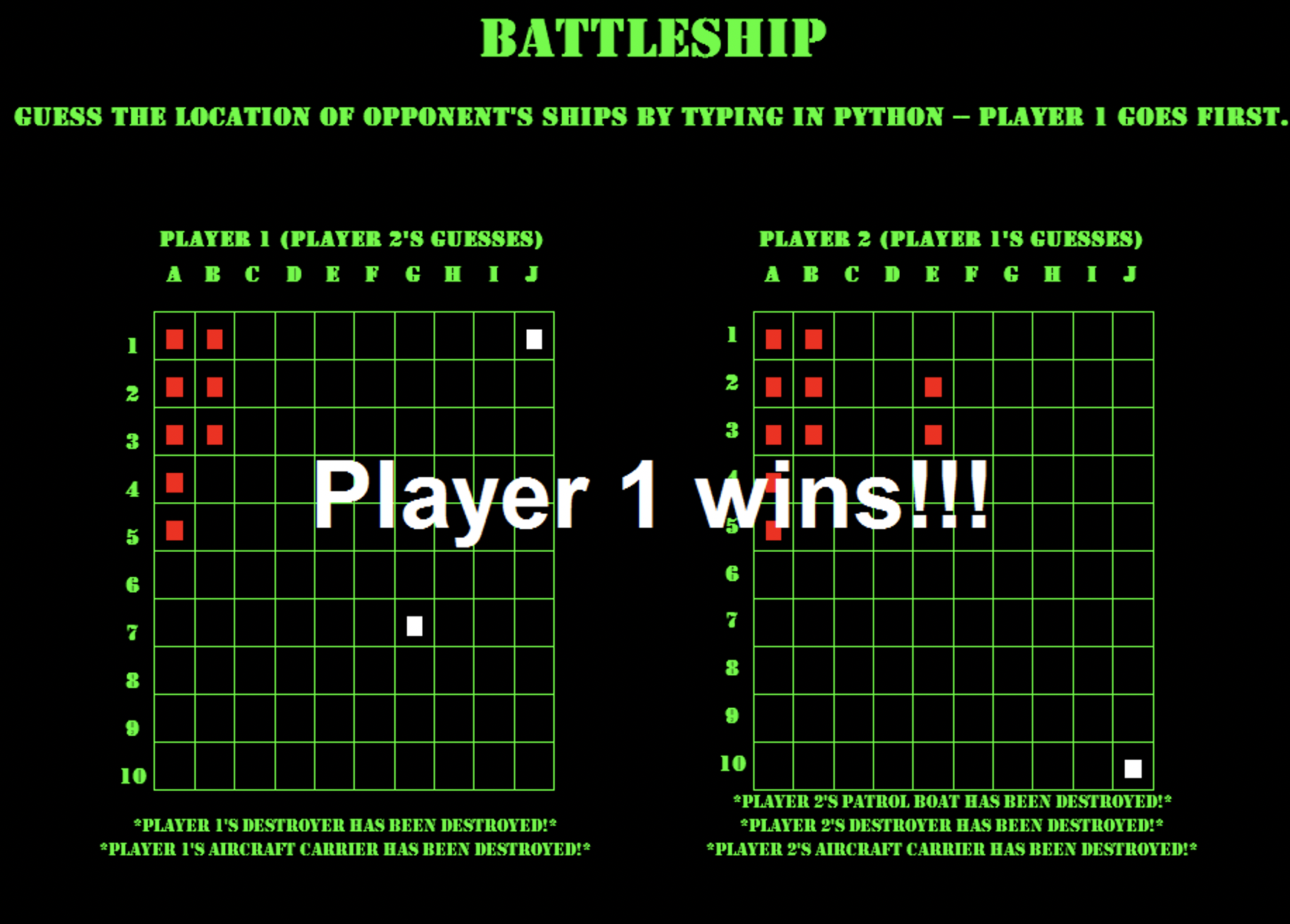
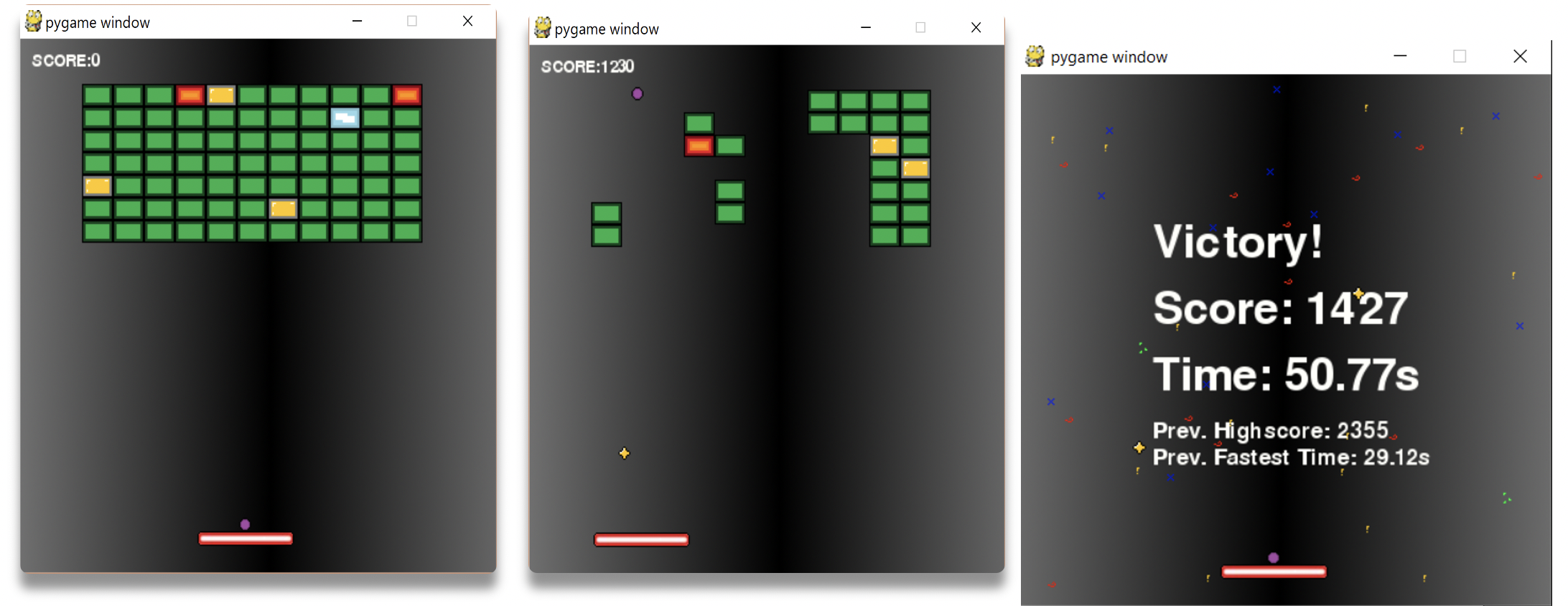
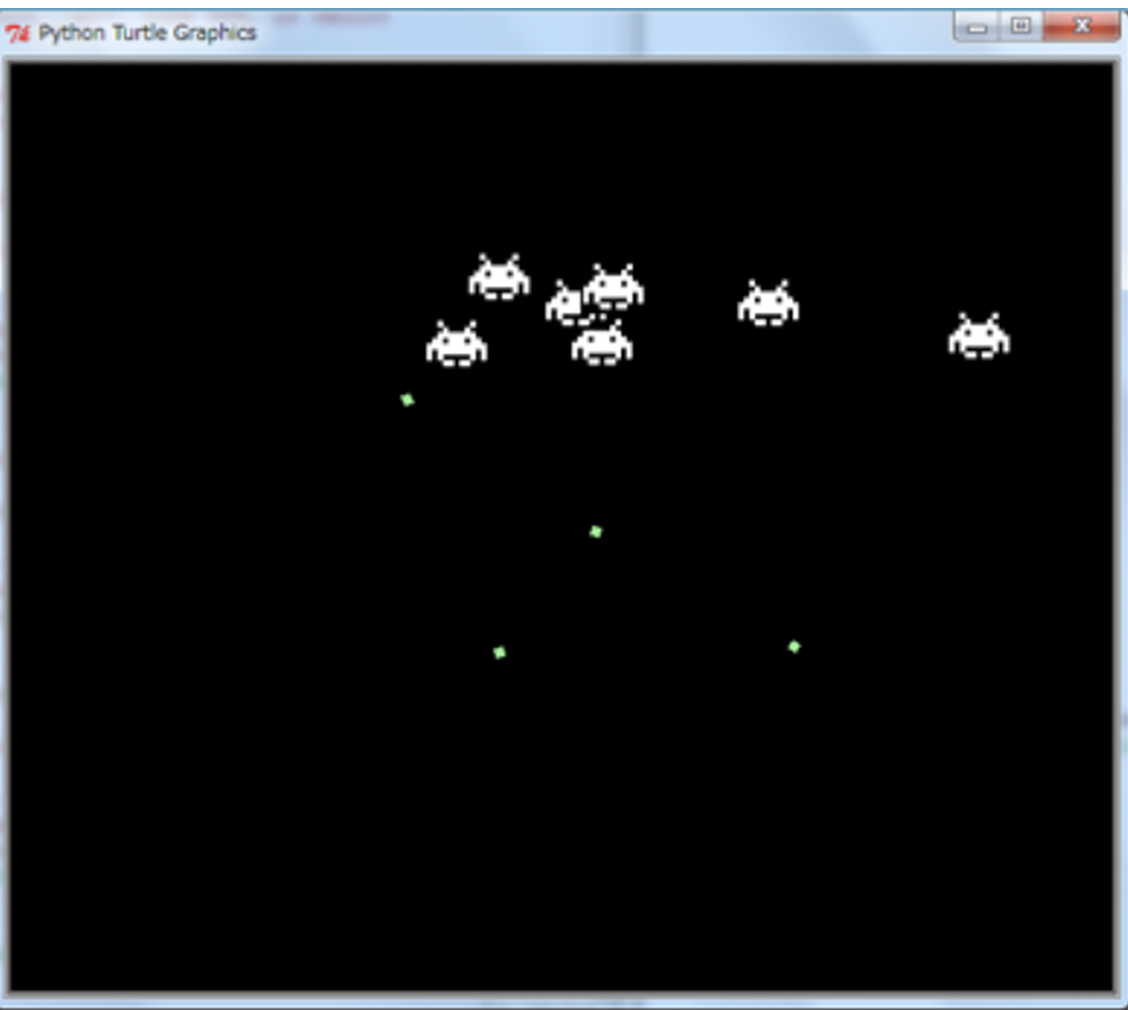
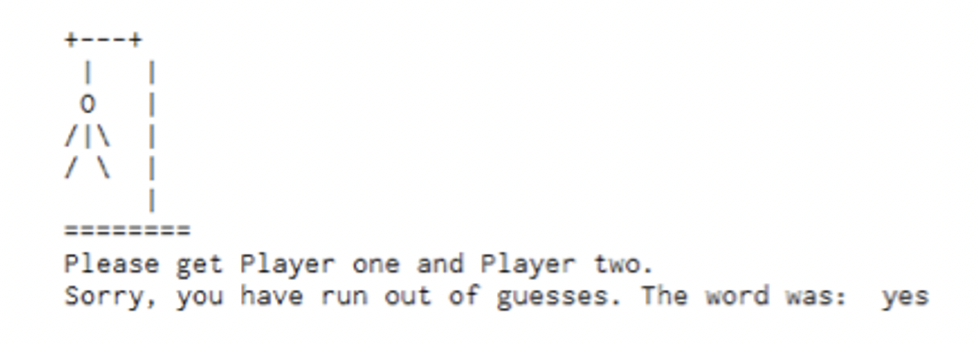
Encryption with images (image contains a secret message embedded within the pixel differences)
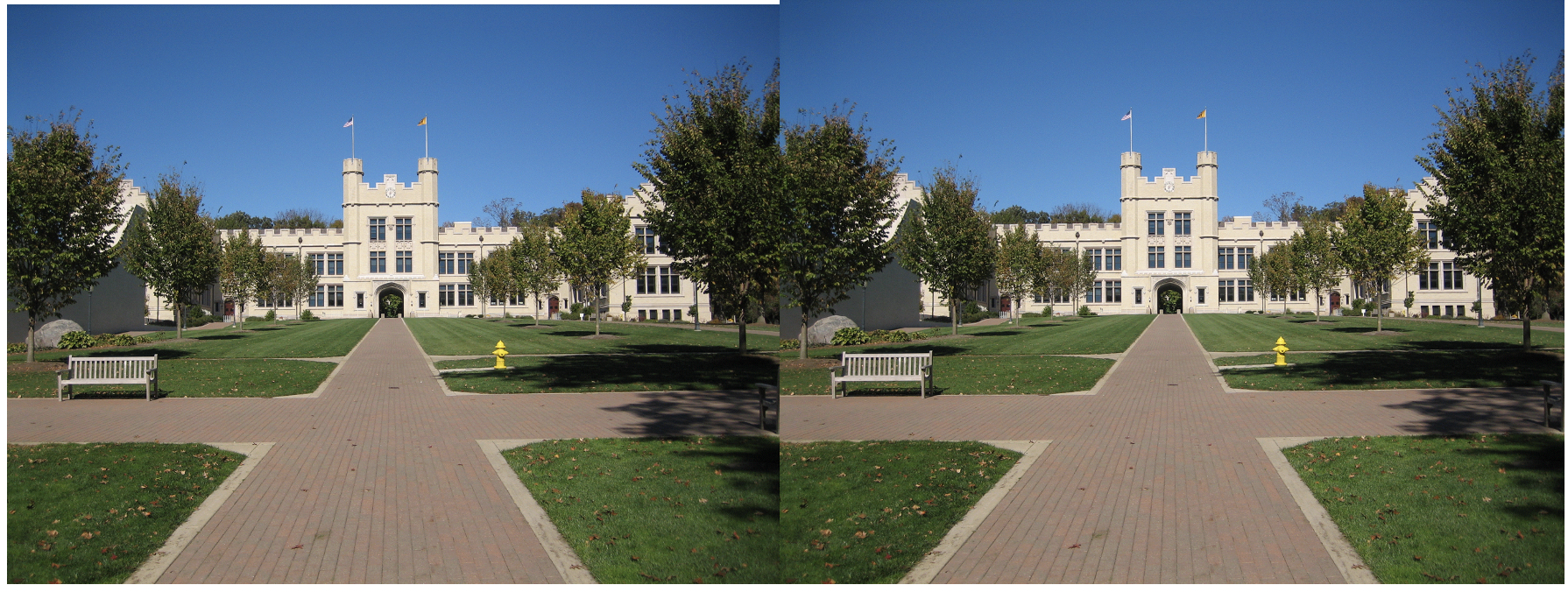
Image processing
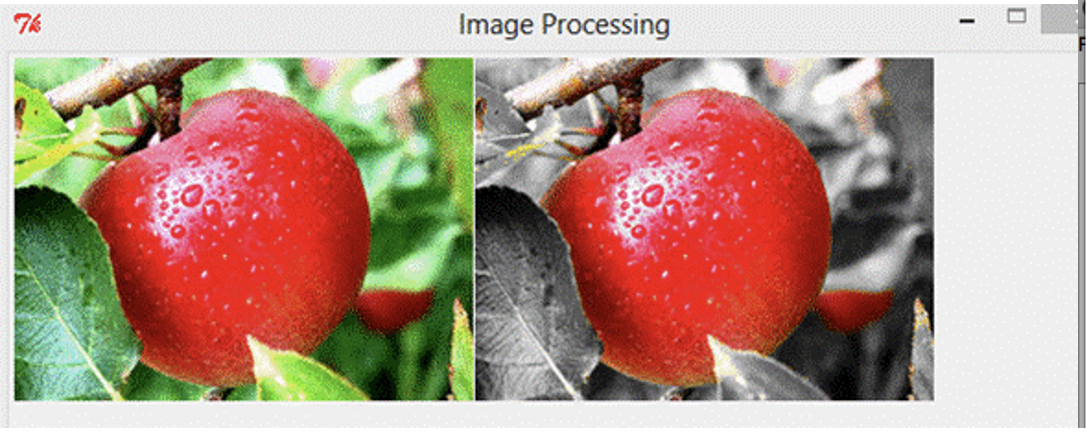
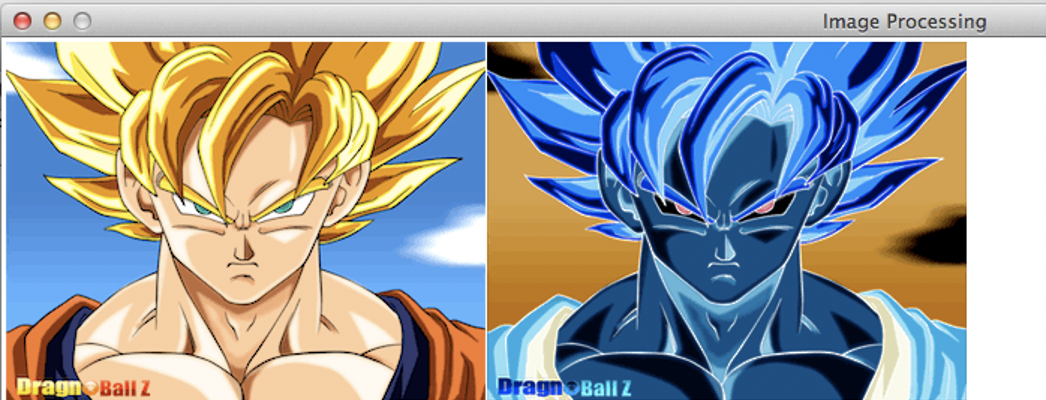
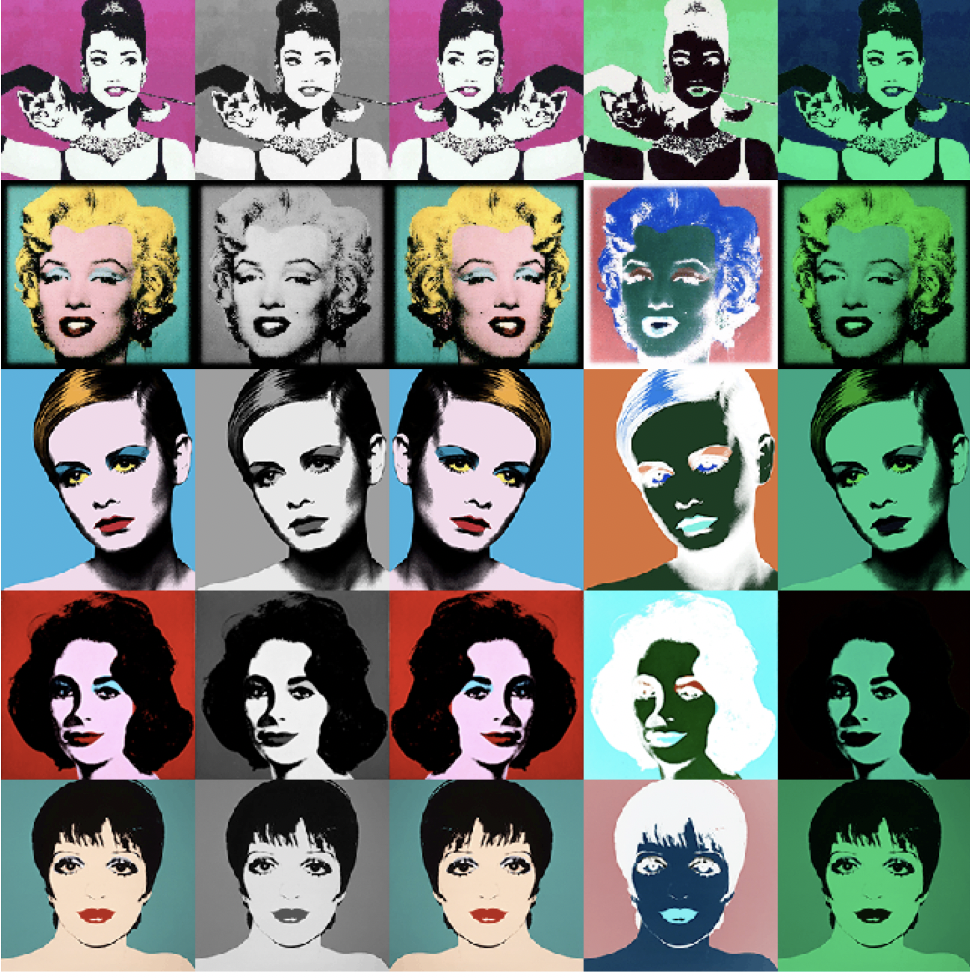
Landscapes
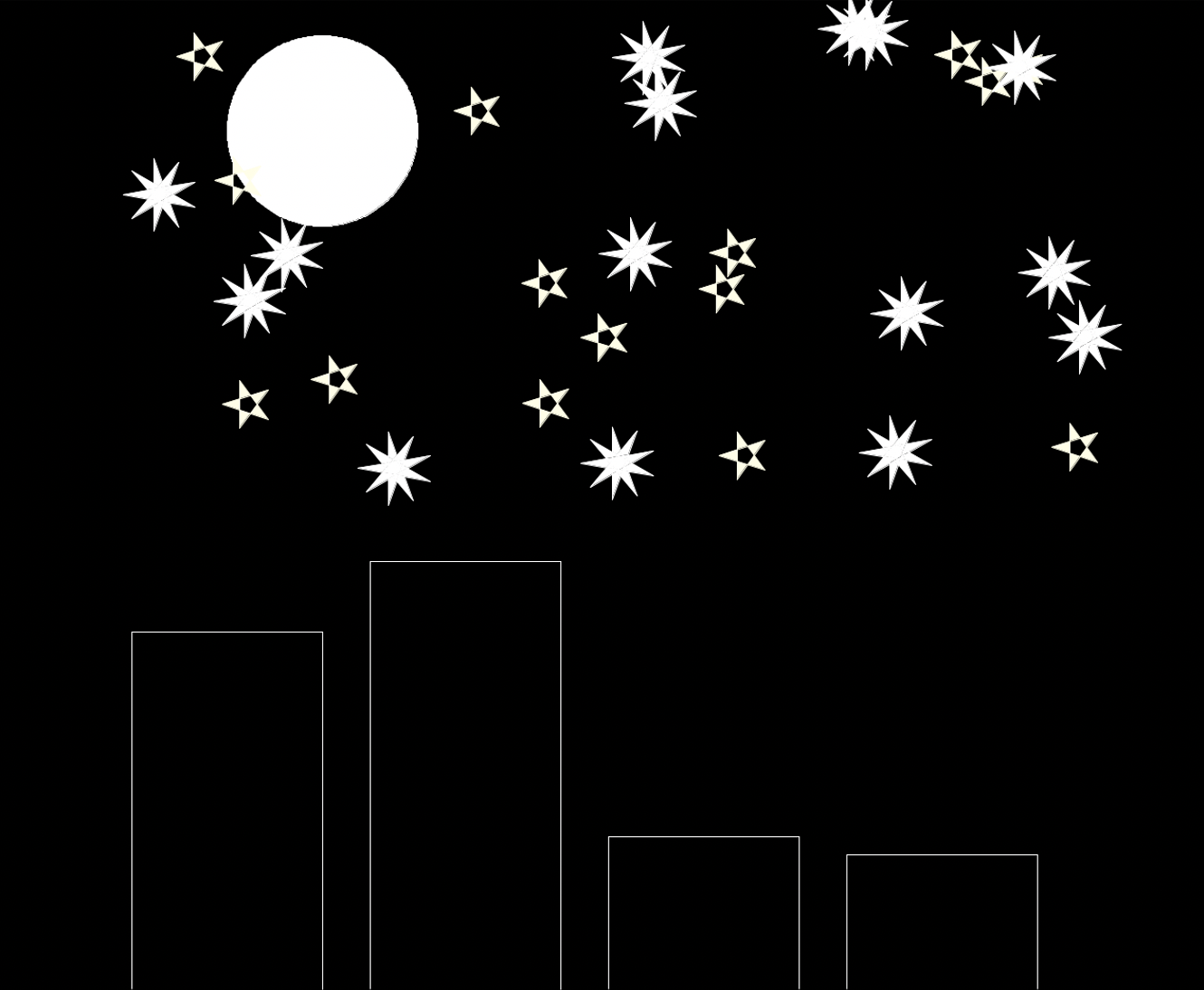
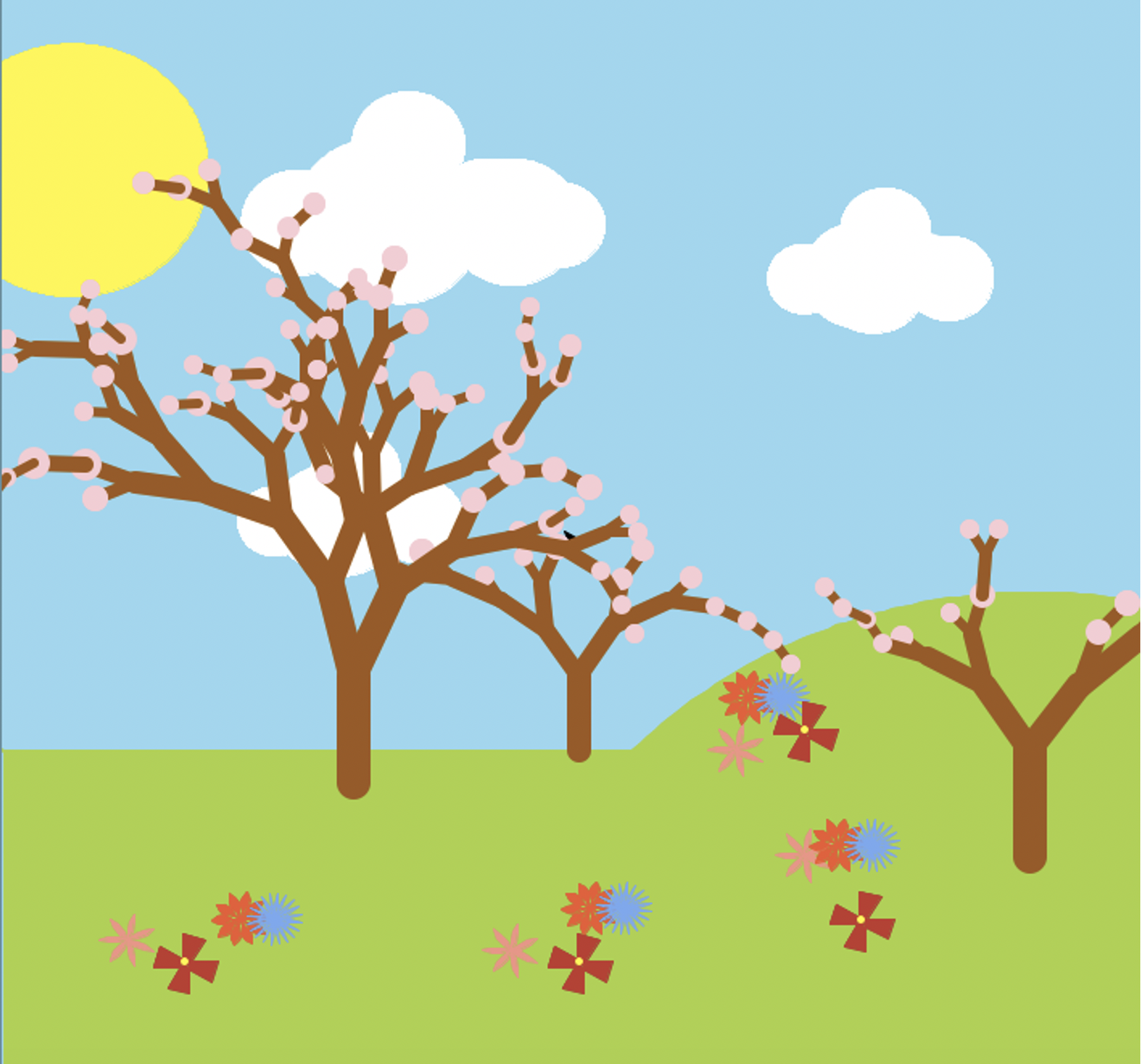
Images created by recursive functions
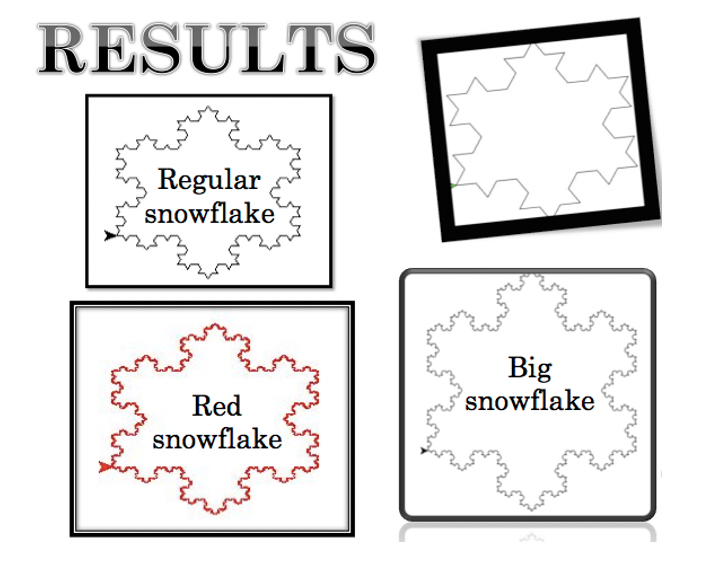
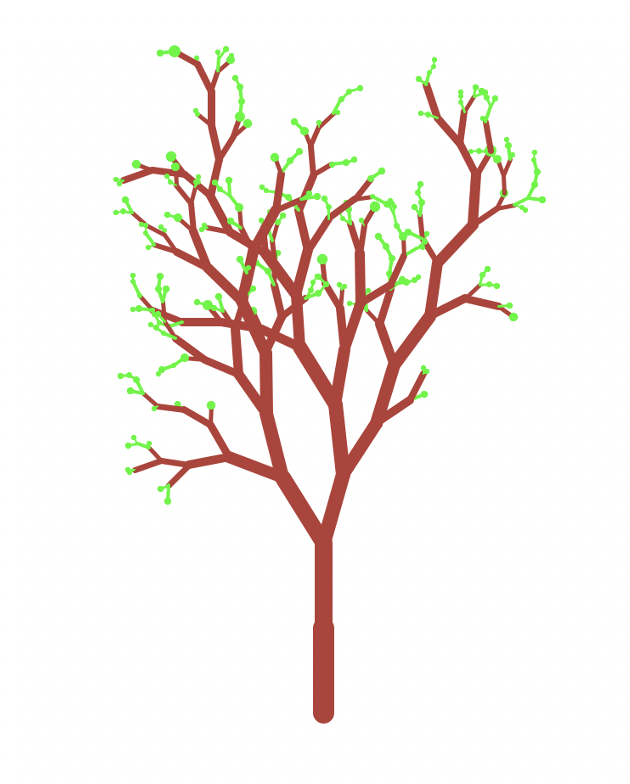
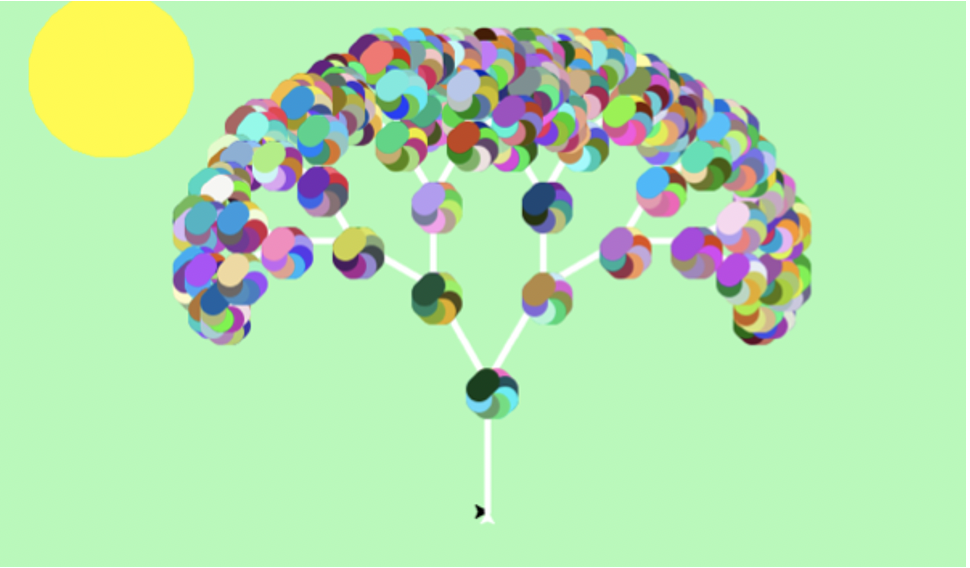
Solar system
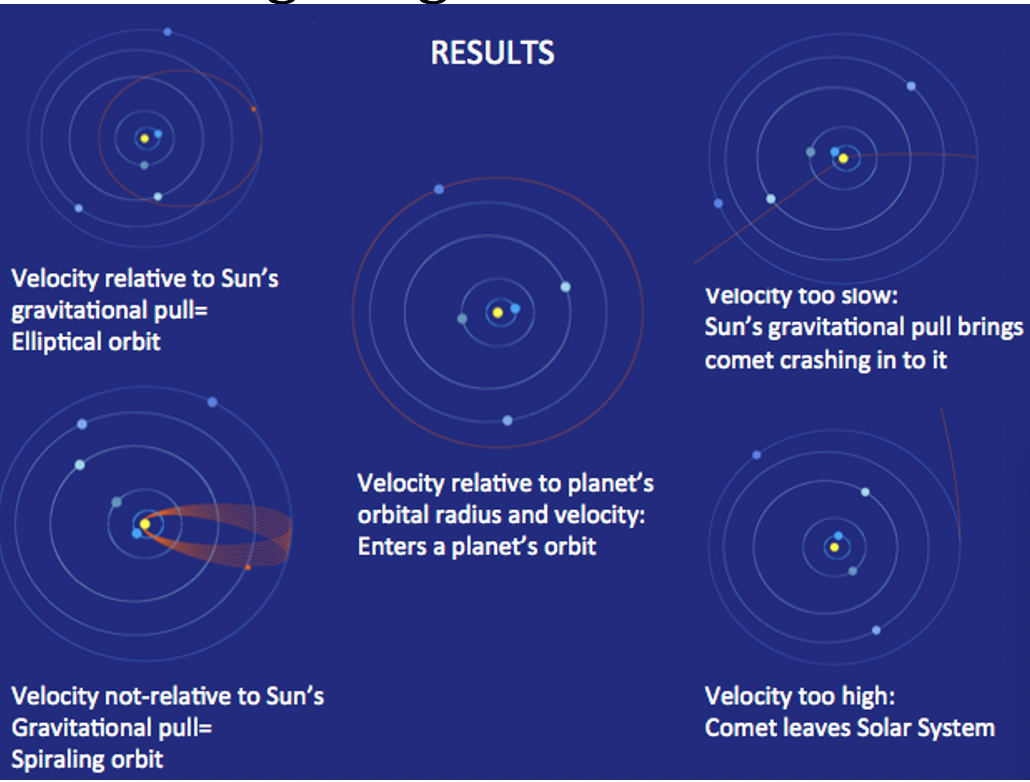
Charts / visualizations
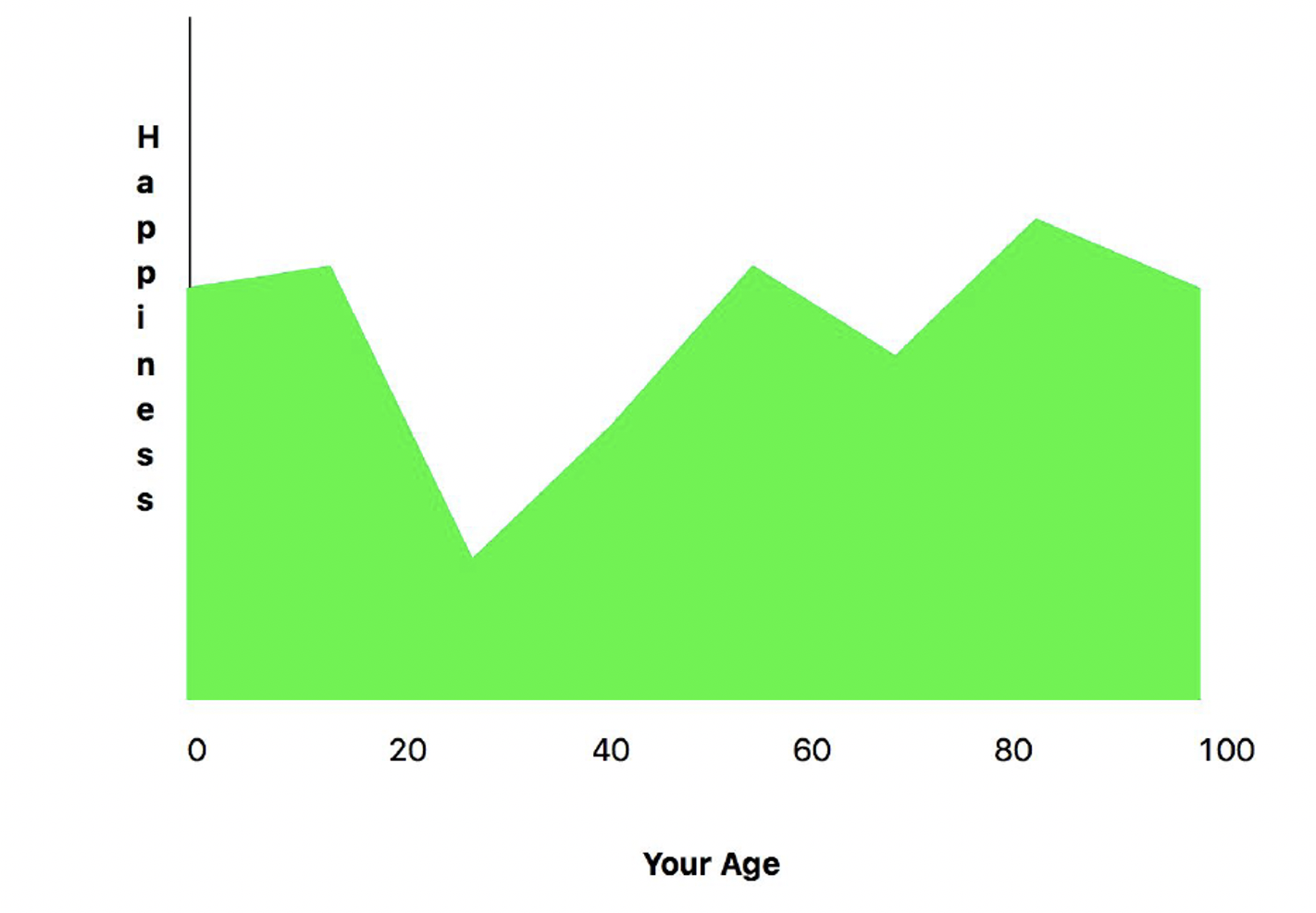